In this article, we are going to learn how to POST XML Data to ASP.NET Core WEB API Using HttpClient from the .Net Core Console Application in simple steps.
#DOTNETSHORTS Series

Nowadays everyone uses the Json format right still we have many legacy applications which use XML for that purpose I have written this article.
If you Like this Article Please comment below🙏.
In previous articles, we have checked how to create and get data in simple steps below are the article’s links.
How to Consume WEB API GET Method in .Net Core Console Application
How to Consume WEB API GET Method with a parameter named id in .Net Core Console Application
How to POST DATA to ASP.NET CORE WEB API Using HttpClient from .Net Core Console Application
Let’s Get Started. 🤩
API which we are going to call

Let’s first call POST API using Postman
URI: – https://localhost:44373/api/Music
We have URI we will use the below XML data to POST data.
<?xml version="1.0" encoding="utf-8"?>
<SongsRequestViewModel
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xmlns:xsd="http://www.w3.org/2001/XMLSchema">
<Name>Oo Antava..Oo Oo Antava</Name>
<Length>3.48</Length>
<Yearreleased>2021</Yearreleased>
<Writer>Chandrabose</Writer>
<Singer>Devi Sri Prasad</Singer>
<Genre>Indian pop</Genre>
<Rating>5</Rating>
</SongsRequestViewModel>
We have set the setting header Content-Type: – application/xml in POSTMAN to send the request.
In response, we will get the same XML object which we have sent for that we need to set Accept Header:- application/xml and in return response, we will get a new parameter in response songid.
Simple Tips
Header parameters: “Accept” vs “Content-type”
Accept header is used by HTTP clients to tell the server which type of content they expect/prefer as a response.
Content type can be used both by clients and servers to identify the format of the data in their request (client) or response (server) and, therefore, help the other part interpret correctly the information.
More Details Information is below.

Creating Console Application
For Consuming APIs, we have created a .Net Core Application which targets Framework .Net 6.0 with the name Consoleconsumer.
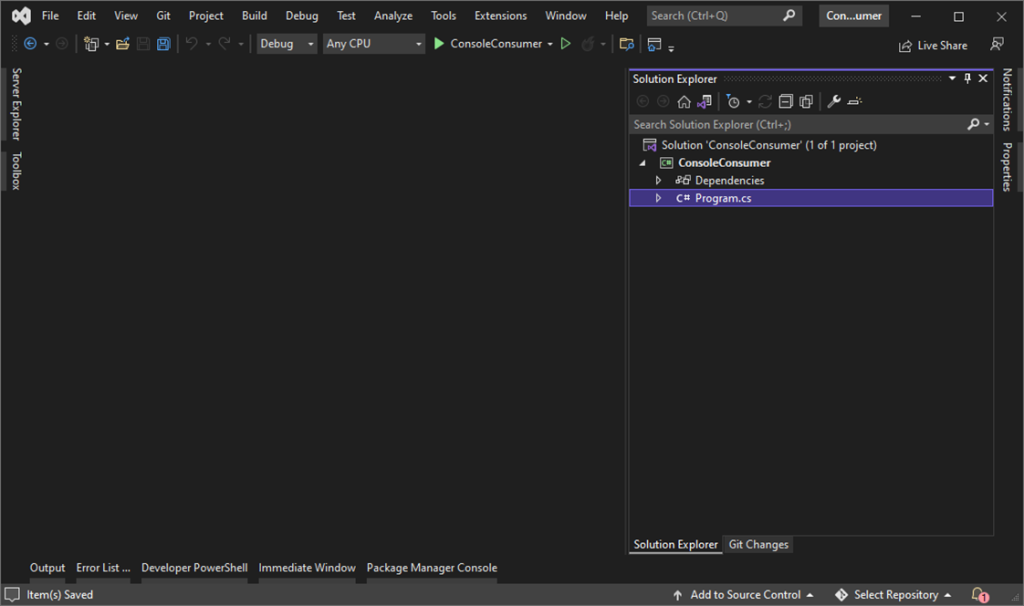
Steps to POST XML data to Web API
- HttpClient class instance for sending HTTP Requests.
- Setting Base Address “https://localhost:44373”
- Setting Accept Header “application/xml”
- Setting Content-Type “application/xml”
- Adding class SongsRequestViewModel which has properties and fields of request API.
- Adding a class with the name SongsResponseModel which has properties and fields of response XML. SongsResponseModel class will be used for the POST object along with it we are also going to use it while deserializing response.
- Next, we are calling PostAsync Method and Passing Request Uri (“/api/Music”) and also HttpContent as a parameter to it which will send a POST request to the specified Uri in an asynchronous way.
- DeserializeObject (response we are going to receive will be Json format which we need to map to .Net Type)
Method for POSTING XML to WEB API
In this method, we are going to first create an instance of HTTPclient.
Now if you see Music POST API, we need POST data for that we need to add a class with similar properties and fields of API with takes input.
SongsRequestViewModel Class
We are going to assign properties to this class and then serialize it to XML. This XML we are going to POST to API.
public class SongsRequestViewModel
{
public string Name { get; set; }
public string Length { get; set; }
public int Yearreleased { get; set; }
public string Writer { get; set; }
public string Singer { get; set; }
public string Genre { get; set; }
public int Rating { get; set; }
}
SongsResponseModel Class
This class is decorated with XML attributes which will be used for deserializing responses which we are going to receive.
[XmlRoot(ElementName = "SongsModel")]
public class SongsResponseModel
{
[XmlElement(ElementName = "SongId")]
public int? SongId { get; set; }
[XmlElement(ElementName = "Name")]
public string Name { get; set; }
[XmlElement(ElementName = "Length")]
public string Length { get; set; }
[XmlElement(ElementName = "Yearreleased")]
public int? Yearreleased { get; set; }
[XmlElement(ElementName = "Writer")]
public string Writer { get; set; }
[XmlElement(ElementName = "Singer")]
public string Singer { get; set; }
[XmlElement(ElementName = "Genre")]
public string Genre { get; set; }
[XmlElement(ElementName = "Rating")]
public int? Rating { get; set; }
}
After adding the request and response classes next we are going to create an instance of a SongsRequestViewModel Class and set some hardcode data to it for the demo.
var songsRequestViewModel = new SongsRequestViewModel()
{
Name = "Oo Antava Oo Oo Antava",
Rating = 5,
Length = "3.48",
Genre = "Indian pop",
Singer = "Devi Sri Prasad",
Writer = "Chandrabose",
Yearreleased = 2021
};
Next after assigning data to SongsRequestViewModel class, we are going to create an instance HttpRequestMessage and then pass HttpMethod Type and RequestURI.
Creating an instance of HttpRequestMessage
var request = new HttpRequestMessage(HttpMethod.Post, "https://localhost:44373/api/Music");
Creating an instance of HttpRequestMessage of string content and pass content, encoding type and content type.
Setting Accept Header
Accept header is used by HTTP clients to tell the server which type of content they expect/prefer as a response.
// Accept Header is set to get response in XML format
client.DefaultRequestHeaders.Add("Accept", "application/xml");
Serializing SongsRequestViewModel object to XML
string xml = "";
XmlSerializer serializer = new XmlSerializer(typeof(SongsRequestViewModel));
await using (var stringWriter = new Utf8StringWriter())
{
await using (XmlWriter writer = XmlWriter.Create(stringWriter, new XmlWriterSettings() { Async = true }))
{
serializer.Serialize(writer, songsRequestViewModel);
xml = stringWriter.ToString(); // Your XML
}
}
Next after assigning data to the class, we are going to create an instance of string content and pass content, encoding type and content type.
HttpContent body = new StringContent(xml, Encoding.UTF8, "application/xml");
In this instance of string content, we are going to pass to PostAsync() method as an input parameter and also, we are going to set request Request-URI as “/api/Music”.
var response = client.PostAsync("https://localhost:44373/api/Music", body).Result;
As the PostAsync method gets called we are going to receive a response and, in this response, the first thing we are going to do is check the Status of it if it is a success then we are going to read the response and then Deserialize it.
The response that we are going to get is the same as what we have sent one different parameter we are going to get is the Key (Primary key) to indicate data is saved in the database successfully.
// Check Response Status Code
if (response.IsSuccessStatusCode)
{
var content = await response.Content.ReadAsStringAsync();
if (!string.IsNullOrEmpty(content))
{
// Deserializing Response from XML to Object
XmlSerializer responseserializer = new XmlSerializer(typeof(SongsResponseModel));
using (StringReader reader = new StringReader(content))
{
var responsesong = (SongsResponseModel)responseserializer.Deserialize(reader);
}
}
}
Complete Source Code
static async Task PostXMLData()
{
using var client = new HttpClient();
var songsRequestViewModel = new SongsRequestViewModel()
{
Name = "Oo Antava Oo Oo Antava",
Rating = 5,
Length = "3.48",
Genre = "Indian pop",
Singer = "Devi Sri Prasad",
Writer = "Chandrabose",
Yearreleased = 2021
};
// Accept Header is set to get response in XML format
client.DefaultRequestHeaders.Add("Accept", "application/xml");
string xml = "";
XmlSerializer serializer = new XmlSerializer(typeof(SongsRequestViewModel));
await using (var stringWriter = new Utf8StringWriter())
{
await using (XmlWriter writer = XmlWriter.Create(stringWriter, new XmlWriterSettings() { Async = true }))
{
serializer.Serialize(writer, songsRequestViewModel);
xml = stringWriter.ToString(); // Your XML
}
}
HttpContent body = new StringContent(xml, Encoding.UTF8, "application/xml");
var response = client.PostAsync("https://localhost:44373/api/Music", body).Result;
// Check Response Status Code
if (response.IsSuccessStatusCode)
{
var content = await response.Content.ReadAsStringAsync();
if (!string.IsNullOrEmpty(content))
{
// Deserializing Response from XML to Object
XmlSerializer responseserializer = new XmlSerializer(typeof(SongsResponseModel));
using (StringReader reader = new StringReader(content))
{
var responsesong = (SongsResponseModel)responseserializer.Deserialize(reader);
}
}
}
}
Calling PostXMLData Method in Main() Method
static void Main(string[] args)
{
PostXMLData().Wait();
}
Snapshots of runtime request and response
While sending a POST request.

Below snapshot of POST API which shows populated parameters
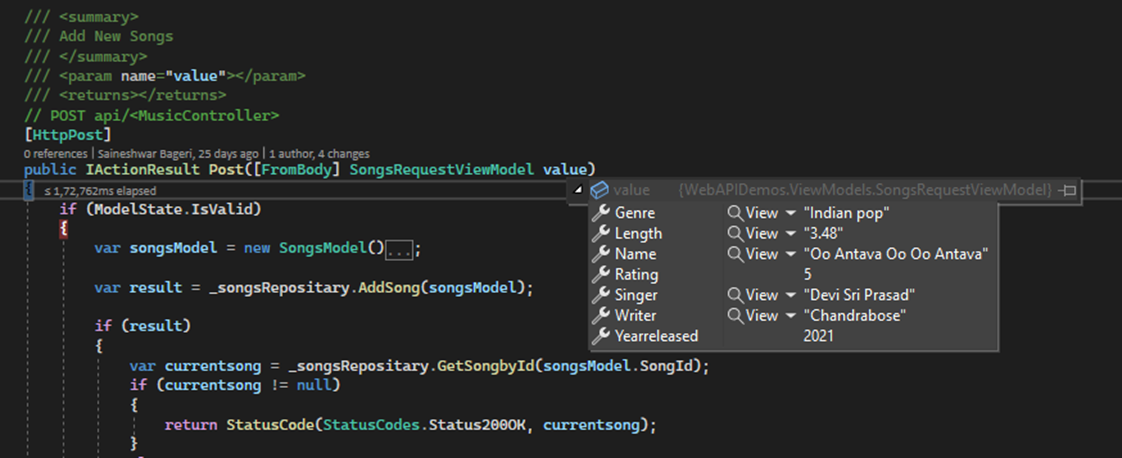
Below snapshot after the deserializing XML object

Hope you have liked it.
In this article, we have learned how to POST XML Data to ASP.NET Core WEB API Using HttpClient from the .Net Core Console Application in simple steps.
Simple Tips
Header parameters: “Accept” and “Content-type” in a REST context
Accept header is used by HTTP clients to tell the server which type of content they expect/prefer as a response.
Content type can be used both by clients and servers to identify the format of the data in their request (client) or response (server) and, therefore, help the other part interpret correctly the information.
More Details Link
Welcome my New Dotnet Shorts #DOTNETSHORTS Series Hope you like it small and simple and focused.